How to use GeometryReader in SwiftUI
Issue #735
From my previous post How to use flexible frame in SwiftUI we know that certain views have different frame behaviors. 2 of them are .overlay
and GeometryReader
that takes up whole size proposed by parent.
By default GeometryReader
takes up whole width and height of parent, and align its content as .topLeading
1 | struct ContentView_Previews: PreviewProvider { |
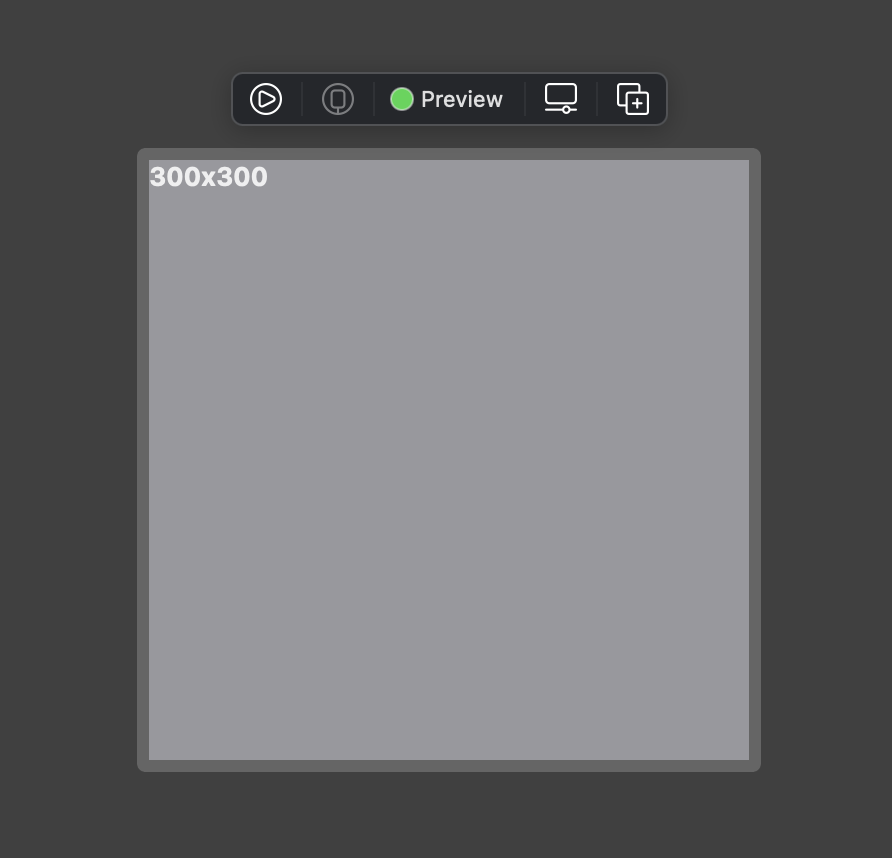
To align content center, we can specify frame with geo
information
1 | struct ContentView_Previews: PreviewProvider { |
The result is that Text is center aligned
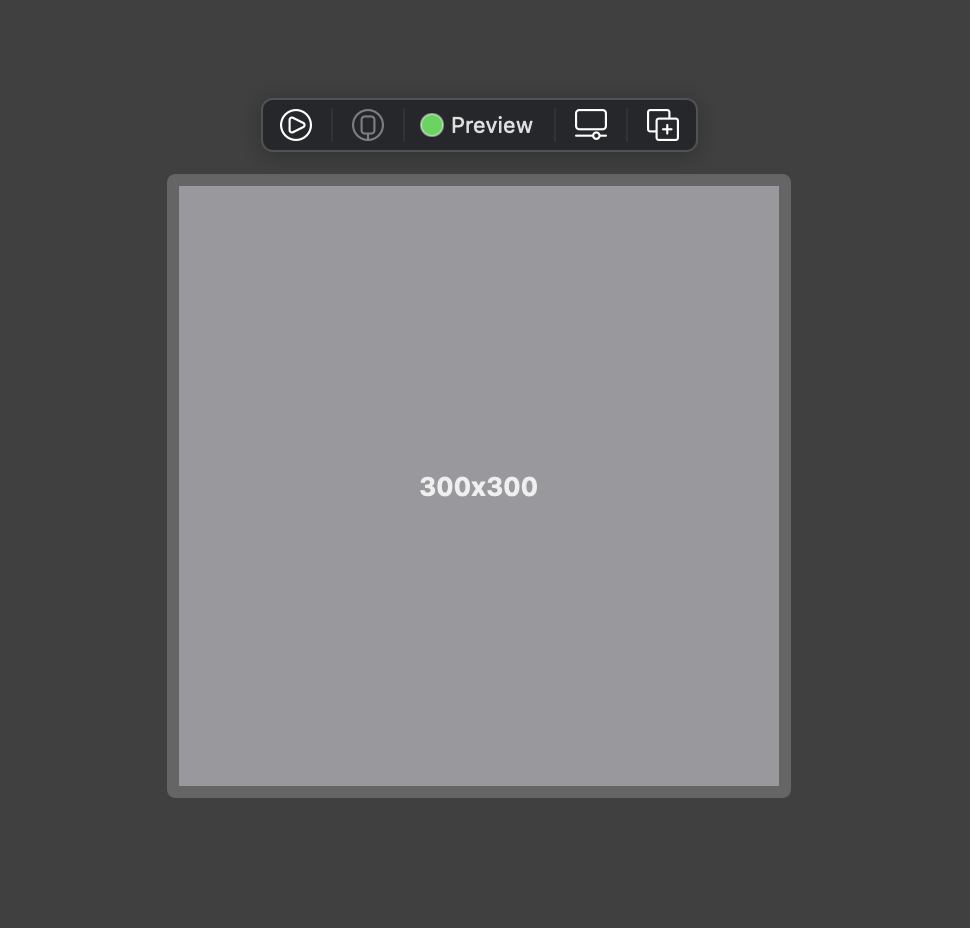
If we were to implement GeometryReader, it would look like this
1 | struct GeometryReader<Content: View>: View { |
Updated at 2021-01-01 23:42:34