Issue #273
Original post https://hackernoon.com/using-bitrise-ci-for-android-apps-fa9c48e301d8
CI, short for Continuous Integration, is a good practice to move fast and confidently where code is integrated into shared repository many times a day. The ability to have pull requests get built, tested and release builds get distributed to testers allows team to verify automated build and identify problems quickly.
I ‘ve been using BuddyBuild for both iOS and Android apps and were very happy with it. The experience from creating new apps and deploying build is awesome. It works so well that Apple acquired it, which then lead to the fact that Android apps are no longer supported and new customers can’t register.
We are one of those who are looking for new alternatives. We’ve been using TravisCI, CircleCI and Jenkins to deploy to Fabric. There is also TeamCity that is promising. But after a quick survey with friends and people, Bitrise is the most recommended. So maybe I should try that.
The thing I like about Bitrise is its wide range support of workflow. They are just scripts that execute certain actions, and most of them are open source. There’s also yml config file, but all things can be done using web interface, so I don’t need to look into pages of documentation just to get the configuration right.
This post is not a promote for Bitrise, it is just about trying and adapting to new things. There is no eternal thing in tech, things come ad go fast. Below are some of the lessons I learn after using Bitrise, hope you find them useful.
Variant to build
There is no Android Build step in the default ‘primary’ workflow, as primary is generally used for testing the code for every push. There is an Android Build step in the deploy workflow and the app gets built by running this workflow. However, I like to have the Android Build step in my primary workflow, so I added it there.
Usually I want app module and stagingRelease build variant as we need to deploy staging builds to internal testers.
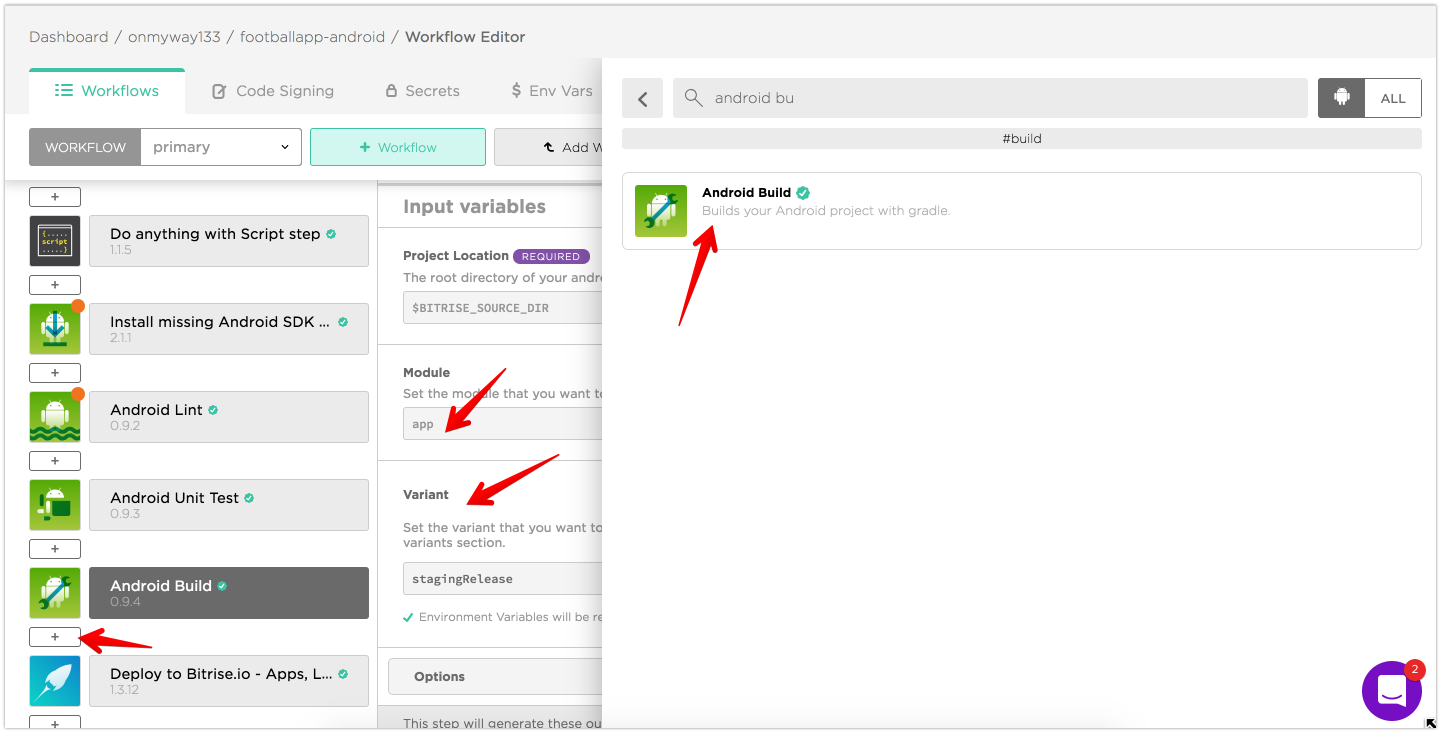
If you go to Bitrise.yml tab you can see that the configuration file has been updated. This is very handy. I’ve used some other CI services and I needed to lookup their documentation on how to make this yml work.
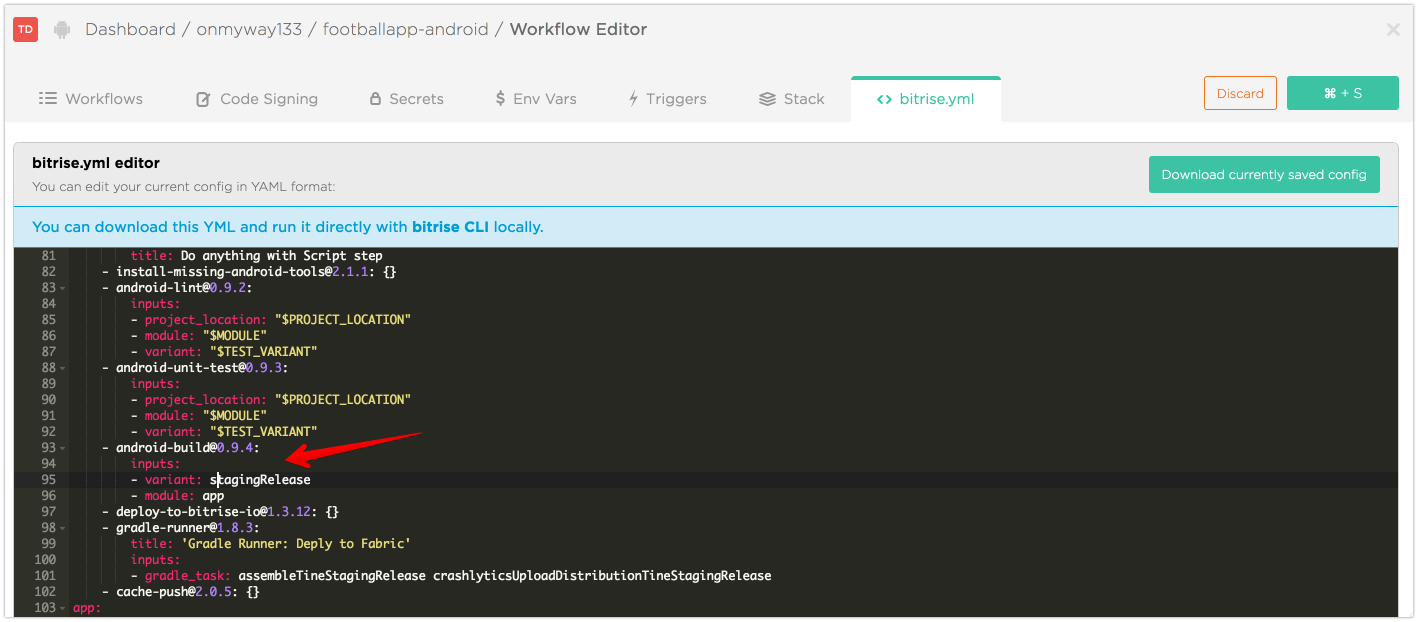
Bump version code automatically
I’ve used some other CI services before and the app version code surely does not start from 0. So it makes sense that Bitrise can auto bump version code from the current number. There are some predefined steps in Workflow but they don’t serve my need
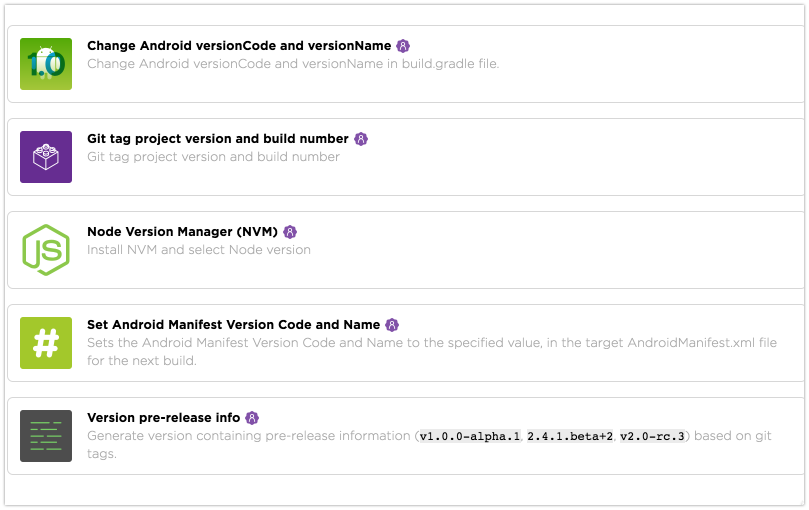
For the Set Android Manifest Version code and name step, the source code is here so I understand what it does. It works by modify AndroidManifest.xml file using sed . This article Adjust your build number is not clear enough.
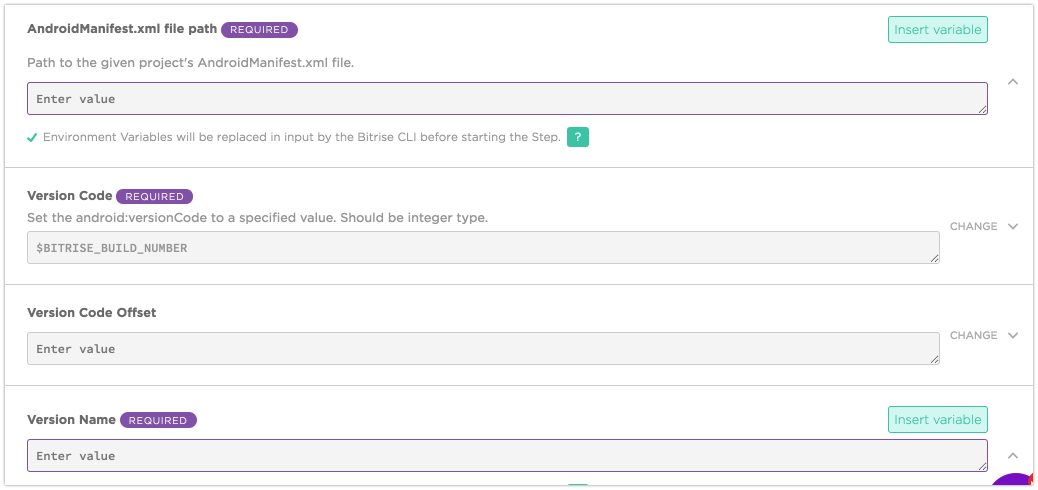
sed -i.bak “s/android:versionCode=”\”${VERSIONCODE}\””/android:versionCode=”\”${CONFIG_new_version_code}\””/” ${manifest_file}
In our projects, the versionCode is from an environment variable BUILD_NUMBER in Jenkins, so we need look up the same thing in Available Environment Variables, and it is BITRISE_BUILD_NUMBER , which is a build number of the build on bitrise.io.
This is how versionCode looks like in build.gradle
versionCode (System.*getenv*("BITRISE_BUILD_NUMBER") as Integer ?: System.*getenv*("BUILD_NUMBER") as Integer ?: 243)
243 is the current version code of this project, so let’s go to app’s Settings and change Your next build number will be
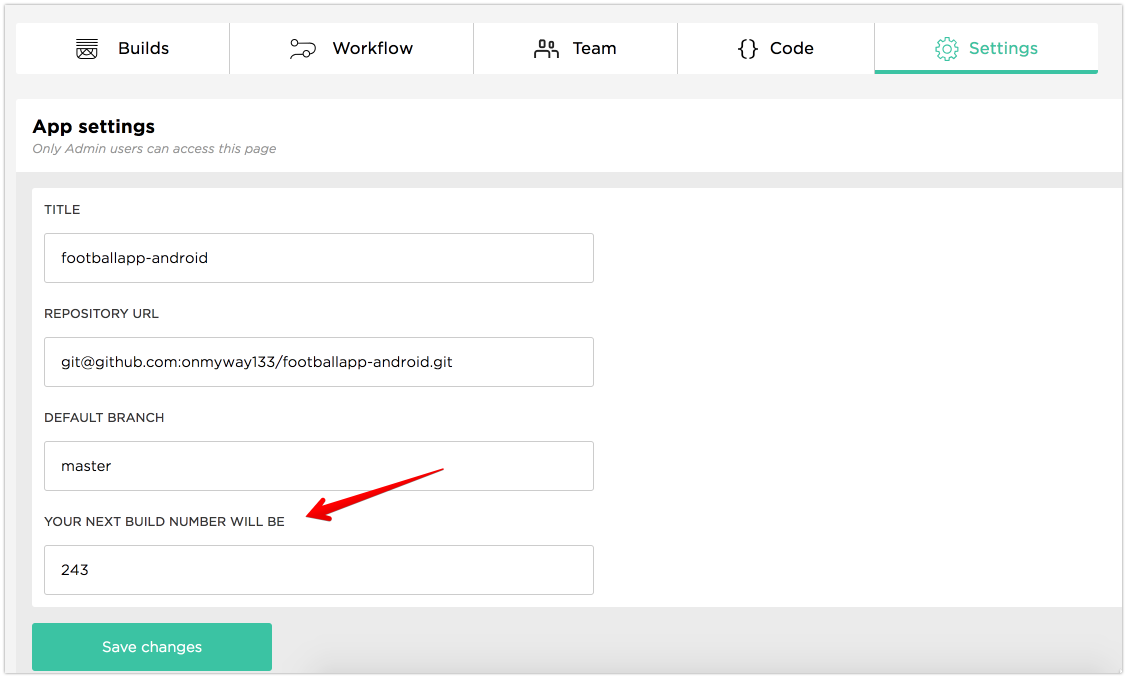
Deploy to Fabric
I hope Bitrise has its own Crash reporting tool. For now I use Crashlytics in Fabric. And despite that Bitrise can distribute builds to testers, I still need to cross deploy to Fabric for historial reasons.
There is only script steps-fabric-crashlytics-beta-deploy to deploy IPA file for iOS apps, so we need something for Android. Fortunately I can use the Fabric plugin for gradle.
Add Fabric plugin
Follow Install Crashlytics via Gradle to add Fabric plugin. Basically you need to add these dependencies to your app ‘s build.gradle
buildscript {
repositories {
google()
maven { url 'https://maven.fabric.io/public' }
}
dependencies {
classpath 'io.fabric.tools:gradle:1.+'
}
}
apply plugin: 'io.fabric'
dependencies {
compile('com.crashlytics.sdk.android:crashlytics:2.9.4@aar') {
transitive = true;
}
}
and API credentials in Manifest file
<meta-data
android:name=”io.fabric.ApiKey”
android:value=”67ffdb78ce9cd50af8404c244fa25df01ea2b5bc”
/>
Deploy command
Modern Android Studio usually includes a gradlew execution file in the root of your project. Run ./gradlew tasks for the list of tasks that you app can perform, look for Build tasks that start with assemble . Read more Build your app from the command line
You can execute all the build tasks available to your Android project using the Gradle wrapper command line tool. It’s available as a batch file for Windows (gradlew.bat) and a shell script for Linux and Mac (gradlew.sh), and it’s accessible from the root of each project you create with Android Studio.
For me, I want to deploy staging release build variant, so I run. Check that the build is on Fabric.
./gradlew assembleStagingRelease crashlyticsUploadDistributionStagingRelease
Manage tester group
Go to your app on Fabric.io and create group of testers. Note that alias that is generated for the group
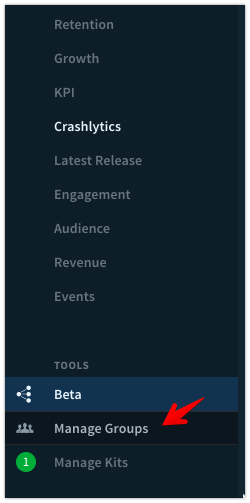
Go to your app’s build.gradle and add ext.betaDistributionGroupAliases=’my-internal-testers’ to your desired productFlavors or buildTypes . For me I add to staging under productFlavors
productFlavors {
staging {
// …
ext.betaDistributionGroupAliases=’hyper-internal-testers-1'
}
production {
// …
}
}
Now that the command is run correctly, let’s add that to Bitrise
Gradle Run step
Go to Workflow tab and add a Gradle Run step and place it below Deploy to Bitrise.io step.
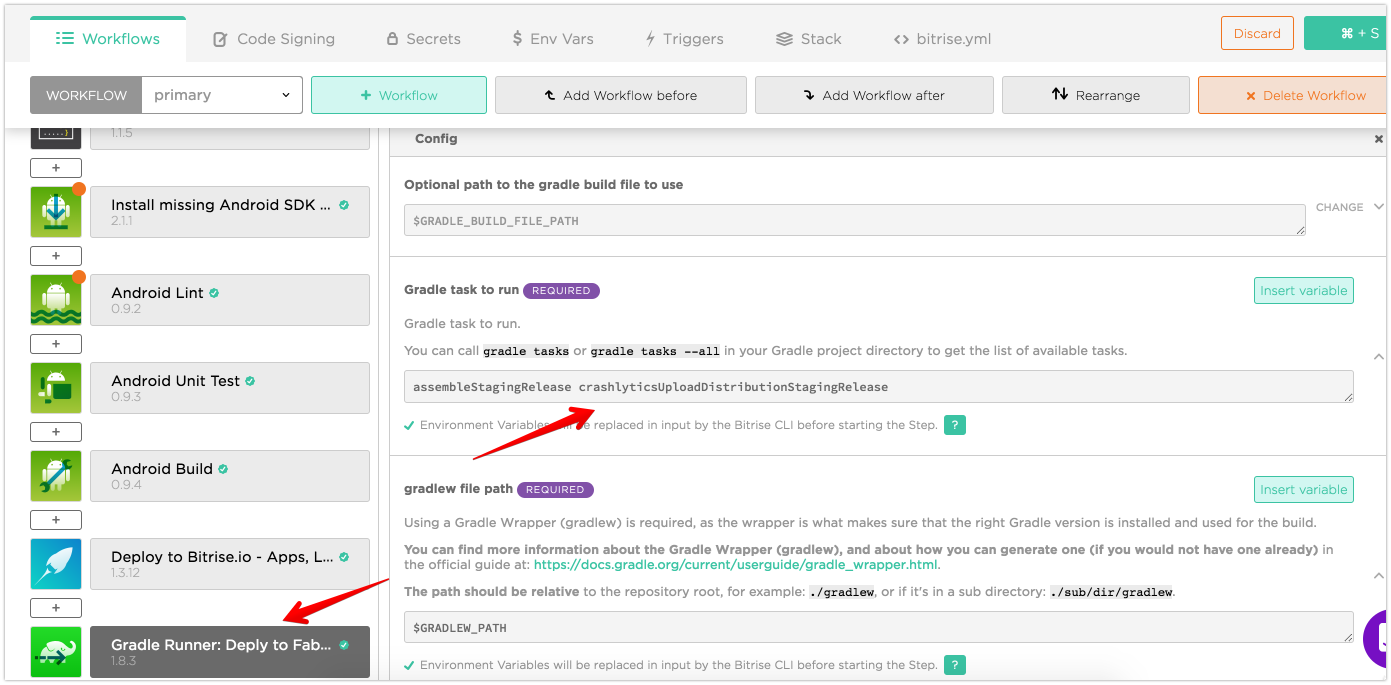
Expand Config, and add assembleStagingRelease crashlyticsUploadDistributionStagingRelease to Gradle task to run .
Now start a new build in Bitrise manually or trigger new build by making pull request, you can see that the version code is increased for every build, crossed build gets deployed to Fabric to your defined tester groups.
As an alternative, you can also use Fabric/Crashlytics deployer, just update config with your apps key and secret found in settings.
Where to go from here
I hope those tips are useful to you. Here are some more links to help you explore further